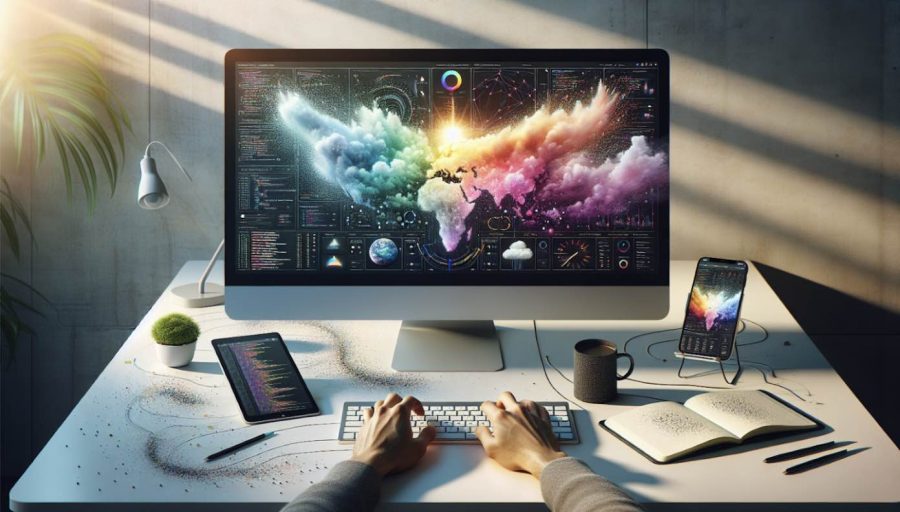
Weather forecast is now something that we all receive regularly and use in many decisions from dressing code to the selection of a time to schedule an event outdoors. The Python weather API has become operational in helping developers acquire meteorological data and come up with applications that give real-time weather updates. This guide is devoted exclusively to integrating the Tomorrow.io weather API with Python so that developers can use current real-time details, temperature, and even weather trends.
Finally, the readers will be guided through this step-by-step tutorial on everything they need to do to get their Python environment ready, successfully perform HTTP calls to the API endpoint, and create a straightforward weather application. Some of the topics included in the guide include; How to obtain an API key, working with JSON data, and visualizing the climate data. By the end of each of the two modules, developers will be able to coordinate Python scripts for purposes of retrieving weather forecasts, historical weather, and even weather alerts with the view of developing applications in fields including but not limited to agriculture, urban planning, and sports, among others.
Setting Up the Python Environment
First of all, let the developers decide on the need to incorporate a Python weather API into their project. This involves installing the necessary libraries and obtaining an API key from Tomorrow.io, a leading provider of meteorological services.
Installing Required Libraries
The first procedure necessary for conducting analysis is to confirm that the Python software is installed on the system. The requirement on the version of Python for this project is Python 2.7.9 or later; or Python 3.4 and later. After getting Python set in its rightful place, the next procedure is getting the needed libraries.
However, to make it easier, there is usually a file created known as the requirements.txt file. This file should list all the necessary libraries for the project, including:
- requests
- flask
- python-dotenv
With the requirements.txt file created, developers can install all the libraries at once using the following command in the terminal or command prompt:
pip install -r requirements.txt
Obtaining an API Key from Tomorrow.io
As for the weather, it is crucial to note that the latter is retrieved from Tomorrow.io in real-time and, therefore, requires having the API key from the company. This key is actually an alphanumeric code, which opens access to a lot of valuable weather information. To obtain an API key:
- The easiest way to retrieve your location data is to go to the Tomorrow.io website and create an account.
- Ensure that the information you give is correct and generate login details.
- Accept the terms of service stated by the provider.
- Click on the Login account and proceed to the API Management tab.
- Next to the “Select Plan” button in the very top right-hand of the page, click ‘Get Your Free API Key’.
- Depending on your feature requirements, go for the Free or start a custom Enterprise plan.
The final elements will be delivered, and you will be able to use your API key. This key can be used to make API calls in different languages like Python, and many others.
As for the API key, it is safer to hide it and place it in a file named .env in the project home directory. Create a file named .env and add the following line:
API_KEY=your_api_key_here
Replace your_api_key_here with the actual API key obtained from Tomorrow.io. By using a .env file, developers can safely share their code without exposing sensitive information.
Making API Requests with Python
Constructing the API Request URL
The first thing that must be done before making an API request is to create the proper URL. For Tomorrow.io, the base URL typically follows this structure:
url = 'https://api.tomorrow.io/v4/timelines'
To make a request, developers have to specify several parameters in the URL of the request, the latter includes the location, the desired weather fields, the units the fields are measured in, and the API key. Here’s an example of how to structure these parameters:
params = {
'location': 'New York',
'fields': 'temperature',
'units': 'imperial',
'apikey': 'your_api_key',
'timesteps': '1h',
'timezone': 'America/New_York',
'endtime': '2023-03-31T12:00:00Z'
}
Sending GET Requests
After designing the URL and the parameters, the next process is to make a GET request to the API URL. The most frequently used method is performing the operation using Python’s requests library. Here’s how to send a GET request:
import requests
response = requests.get(url, params=params)
Parsing the JSON Response
After receiving the response from the API, the next step is to parse the JSON data. Python’s json library is useful for this task. Here’s how to parse the JSON response:
import json
data = json.loads(response.text)
This code converts the JSON response into a Python dictionary, making it easier to extract specific weather information. For example, to retrieve the current temperature:
temperature = data['data']['timelines']['intervals']['values']['temperature']
print(f"The current temperature in New York is {temperature} degrees Fahrenheit.")
Building a Simple Weather Application
Designing an interactive User Interface for a weather app where a user needs to enter the coordinates and get the description of weather: to develop an app using Python and Tomorrow.io weather API requires a simple layout to input location parameters and output weather data. In this section, developers will be shown in detail how to create a basic and usable weather search application.
Creating the User Interface
Designing the user experience of a weather application involves laying down the framework required in using Python and the Tomorrow.io weather API, to input the details of a location and to display the relevant details. In this section, readers will be taken through a step-by-step process of creating a basic but useful application to look up the weather.
Python developers can make use of interfaces from their native applications or third-party application interface requirements. In the case of simple but common needs like building a command line interface, the module argparse appears to be quite valuable. Here’s how to set it up:
import argparse
parser = argparse.ArgumentParser(description="Weather Lookup Tool")
parser.add_argument("city", nargs="+", help="Name of the city")
parser.add_argument("-i", "--imperial", action="store_true", help="Use imperial units")
args = parser.parse_args()
The program below lets a user enter a city name and an optional parameter that indicates whether he/she would like temperature to be displayed in imperial units. Thanks to the nargs=”+” parameter, people can type in the full name of cities for example ‘New York City.
Implementing the Weather Lookup Functionality
Now that we have the User Interface set, the next thing to tackle is the Look-up for the weather using Tomorrow.io API. This involves making HTTP requests to the API endpoint and parsing the JSON response. Here’s a basic implementation:
import requests
import json
def get_weather_data(city, api_key):
url = f"https://api.tomorrow.io/v4/timelines?location={city}&apikey={api_key}"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
return data
else:
return None
# Usage
api_key = "YOUR_API_KEY"
city = " ".join(args.city)
weather_data = get_weather_data(city, api_key)
if weather_data:
temperature = weather_data['data']['timelines']['intervals']['values']['temperature']
description = weather_data['data']['timelines']['intervals']['values']['weatherCode']
print(f"Temperature: {temperature}°C")
print(f"Weather: {description}")
else:
print("Error fetching weather data")
This code itself makes an API call to Tomorrow.io using the requests library, fetches the current temperature and the weather description, and then finally prints to the user. Do not forget to place YOUR_API_KEY as your actual key of the Tomorrow.io API.
When these elements are incorporated they can build a rudimentary Python weather API application that allows a user to search for the current weather of any city. This can go further by allowing more features like forecast, and previous results as well as even a GUI for a more powerful weather application.
Conclusion
The addition of the Tomorrow.io weather API to Python has created a powerful new tool in the weather app development space. This tutorial has outlined how to get the right setting, how to make an API request, and how to create a basic weather search application. Through these steps, the developers are then able to harness loads of meteorological data, which will help them create applications that contain real-time weather information and forecasts.
This technology holds promise in technology, it can be utilized in simple personal weather apps and complex systems that would be used in agricultural or urban planning. In this way, whether APIs are flushed out and developers seek more exciting and constructive ways, more applications like this would surface. This guide is meant to help start and inspire developers to further explore the subject of weather data and build the applications that make a difference in people’s lives.